- A cube that dramatizes the shadow that the user casts upon it. It contains a light sensor, and changes its light output to reflect the amount of light it senses.
- A cube that has likes people but has a limit to the amount of social interaction. It glows until it senses human motion via a passive infrared sensor. After a prolonged period of time with a lot of motion, it "tires out" and "closes up" by becoming very dim.
- A cube that likes to be spoken to softly. Similar to the above, it emits a glow that invites users to approach it. It changes color as it is spoken to. If it is spoken to in a very loud voice, it will become very dim.
3 sensors that can be useful in implementing intangible interaction:
- A force-sensitive resistor can be useful for intangible interactions, as long as it's not measuring the force applied from a user's hands or arms! They can be used to sense weight and to sense whether objects have been placed in a certain area.
- RFID can be used to sense when a tagged object is placed close to the sensor.
- Piezoelectric sensors are used for both microphones and accelerometers. Maybe we can use a microphone to detect movement?
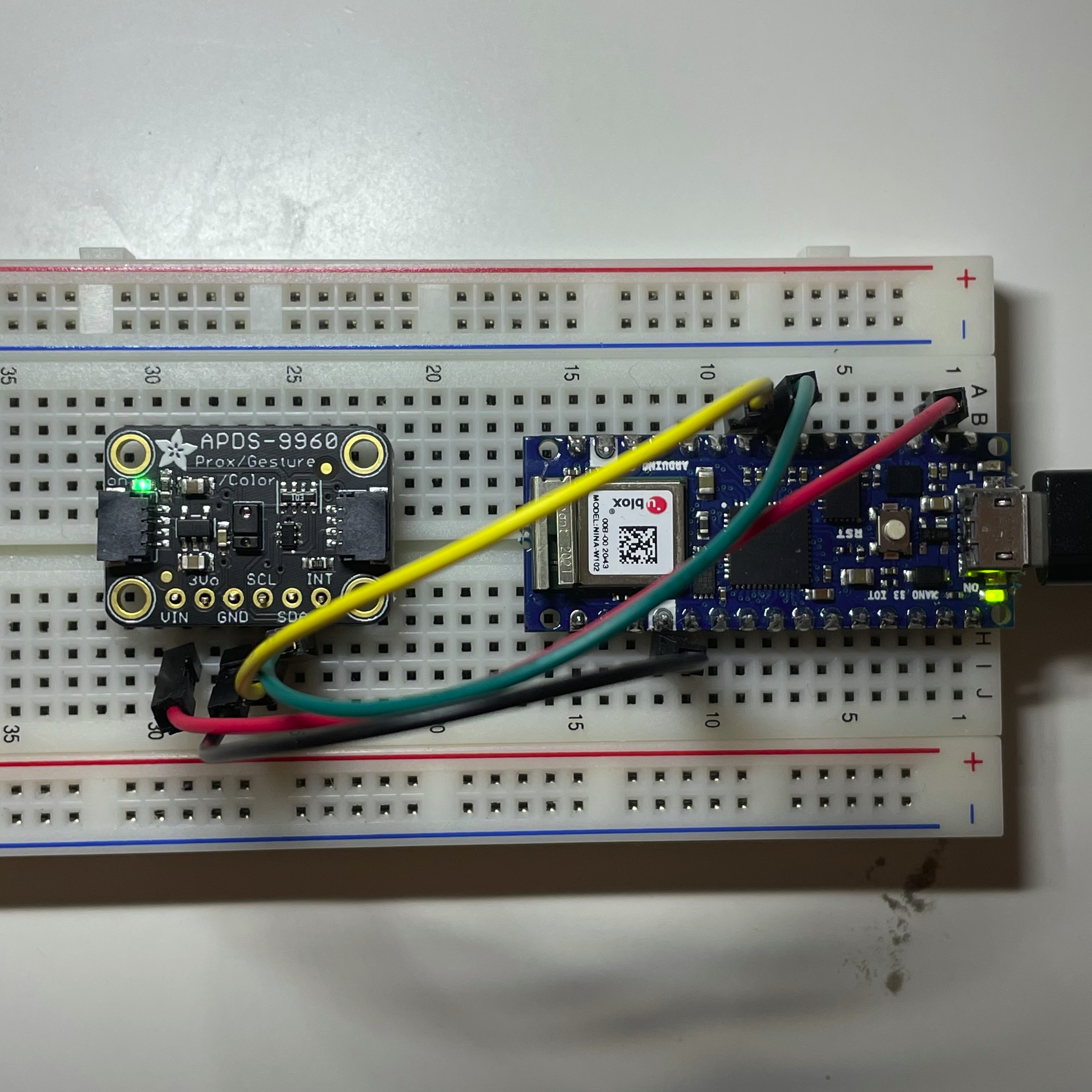
I tried out the ADPS9960 gesture sensor and made a small project with it! The ADPS9960 includes a light and color sensor. I used the light sensor to determine if the room was dark, and changes the color scheme of the computer based to match the room.
Code (Arduino):
Code
#include "Arduino_APDS9960.h" void setup() { Serial.begin(9600); // wait for Serial Monitor to open: while (!Serial) ; Serial.println("hi"); // if the sensor doesn't initialize, let the user know: while (!APDS.begin()) { Serial.println("APDS9960 sensor not working. Check your wiring."); delay(1000); } Serial.println("Sensor is working"); } void loop() { // red, green, blue, clear channels: int r, g, b, c; // if the sensor has a reading: if (APDS.colorAvailable()) { // read the color APDS.readColor(r, g, b, c); // print the values Serial.print(r); Serial.print(","); Serial.print(g); Serial.print(","); Serial.print(b); Serial.print(","); Serial.println(c); delay(100); } }
Code (Computer, Deno + AppleScript):
Code
import { readLines } from "https://deno.land/std@0.77.0/io/bufio.ts"; let lastSetting: boolean | undefined = undefined; async function setDarkMode(setting: boolean) { if (setting === lastSetting) return; lastSetting = setting; let process = await Deno.run({ cmd: [ "osascript", "-e", `tell application "System Events" tell appearance preferences set dark mode to ${setting} end tell end tell`, ], }); await process.status(); } if (import.meta.main) { let process = Deno.run({ cmd: ["make", "monitor"], stdout: "piped", }); for await (let line of readLines(process.stdout)) { let m = line.match(/(\d+),(\d+),(\d+),(\d+)/); if (m) { let [_, sr, sg, sb, sc] = m; let [r, g, b, c] = [sr, sg, sb, sc].map(parseFloat); console.log(c); setDarkMode(c < 45); } else { console.log(line); } } }